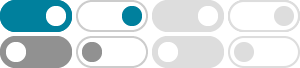
Quick Sort - GeeksforGeeks
Feb 4, 2025 · QuickSort is a divide-and-conquer sorting algorithm that selects a pivot, partitions the array around it, and recursively sorts the resulting sub-arrays.
Quick Sort Algorithm
Quick sort algorithm is often the best choice for sorting because it works efficiently on average O (nlogn) time complexity. It is also one of the best algorithms to learn divide and conquer approach. In this blog, you will learn: 1) How quick sort works? 2) How to choose a good pivot?
algorithm - Quicksort: Iterative or Recursive - Stack Overflow
You can implement an iterative version of Quicksort with a queue rather than a stack. There's nothing about the algorithm that requires the extra storage to be LIFO. The stack approach is more similar to the recursive description commonly used for Quicksort, but that's not actually an inherent part of the algorithm.
13.11. Quicksort — CS201-OpenDSA Data Structures and Algorithms
Jan 7, 2025 · Quicksort is inherently recursive, because each Quicksort operation must sort two sublists. Thus, there is no simple way to turn Quicksort into an iterative algorithm.
QuickSort Algorithm - Algotree
QuickSort is a sorting algorithm based on the divide and conquer strategy. Quick Sort algorithm beings execution by selecting the pivot element, which is usually the last element in the array. The pivot element is compared with each element before placing it in its final position in the array.
Apply Quick Sort Algorithm to sort the list E, X, A, M, P, L, E
Quick Sort is a Recursive algorithm. It divides the given array into two sections using a partitioning element called a pivot. All the elements to the left side of the pivot are smaller than the pivot. All the elements to the right side of the pivot are greater than the pivot.
QuickSort Complete Tutorial | Example | Algorithm - CSEStack
Dec 3, 2023 · How to implement the QuickSort algorithm? The below function can be used as a recursive approach to sort elements using Quick Sort. QuickSort(A,start,end){ if(start >= end){ //calling partition function pIndex <- Partition(A,start,end); QuickSort(A, start, pIndex-1); QuickSort(A,pIndex+1,end); } }
Quick Sort using recursion | MyCareerwise
Quicksort is a sorting algorithm based on the divide and conquer paradigm. In this algorithm the array is divided into two sub-lists, one sub-list will contain the smaller elements and another sub-list will contain the larger elements and then these sub-lists are sorted again using recursion.
Quick Sort Algorithm | CodingDrills
Quick Sort is an efficient sorting algorithm that follows the Divide and Conquer paradigm. It works by partitioning an array into two subarrays, recursively sorting these subarrays, and finally combining them to obtain a sorted array.
c - Binary trees and quicksort? - Stack Overflow
Write a program that sorts a set of numbers by using the Quick Sort method using a binary search tree. The recommended implementation is to use a recursive algorithm.